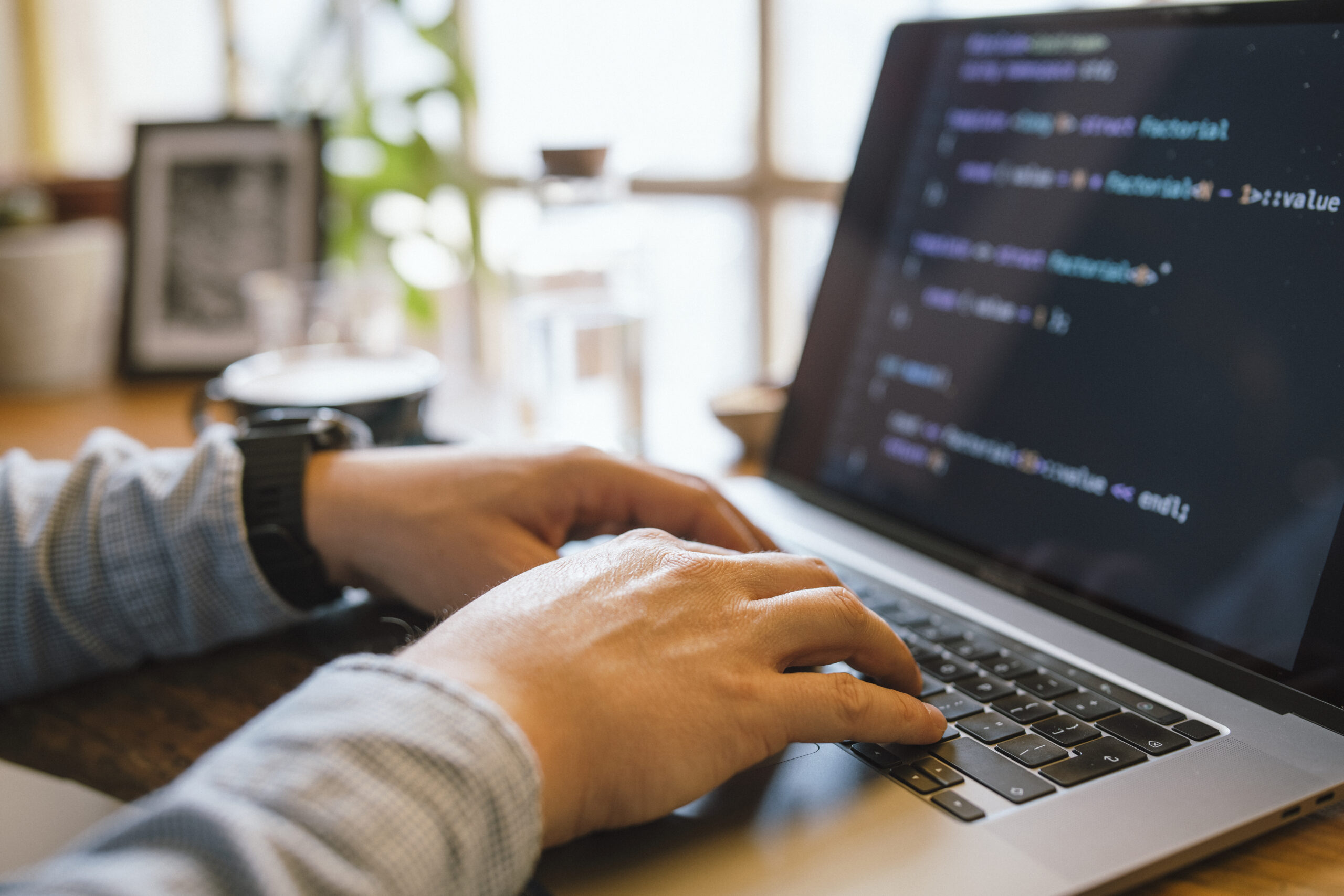
Debugging is Among the most critical — however usually neglected — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to Believe methodically to solve issues effectively. No matter whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of disappointment and drastically boost your productivity. Listed here are a number of strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is a single A part of enhancement, figuring out the way to interact with it correctly through execution is equally critical. Modern-day growth environments arrive Outfitted with effective debugging capabilities — but lots of builders only scratch the surface of what these tools can perform.
Get, by way of example, an Integrated Improvement Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These applications assist you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and even modify code to the fly. When utilised properly, they Permit you to observe accurately how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, check community requests, see true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch disheartening UI concerns into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than managing procedures and memory administration. Studying these equipment can have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Model control methods like Git to comprehend code heritage, come across the precise moment bugs had been introduced, and isolate problematic adjustments.
In the long run, mastering your instruments usually means going past default settings and shortcuts — it’s about building an intimate understanding of your growth natural environment to make sure that when issues arise, you’re not lost in the dark. The better you realize your applications, the greater time you could spend resolving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the condition
One of the more significant — and infrequently forgotten — methods in powerful debugging is reproducing the challenge. Just before jumping into your code or earning guesses, builders want to create a consistent ecosystem or circumstance the place the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of likelihood, often bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire thoughts like: What actions led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it becomes to isolate the precise circumstances underneath which the bug occurs.
As you’ve collected plenty of details, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration producing automated exams that replicate the sting instances or condition transitions associated. These tests not simply help expose the trouble and also prevent regressions Later on.
From time to time, the issue could be natural environment-specific — it might come about only on selected operating techniques, browsers, or underneath individual configurations. Utilizing resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mentality. It requires persistence, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're already halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages are frequently the most precious clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications from the procedure. They usually let you know precisely what transpired, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Commence by studying the information thoroughly and in comprehensive. A lot of developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — go through and understand them 1st.
Break the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Discovering to recognize these can substantially speed up your debugging approach.
Some faults are vague or generic, As well as in Those people circumstances, it’s important to examine the context during which the mistake happened. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater difficulties and supply hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When utilized efficiently, it provides actual-time insights into how an application behaves, assisting you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts all through progress, Data for basic activities (like effective start-ups), Alert for likely concerns that don’t break the applying, Mistake for real issues, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical activities, state improvements, input/output values, and important determination points in the code.
Format your log messages Evidently and persistently. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting This system. They’re Specifically important in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Which has a effectively-considered-out logging approach, it is possible to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly detect and fix bugs, developers need to tactic the procedure similar to a detective solving a mystery. This attitude can help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis bring about.
Start out by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a criminal offense scene, gather as much related details as you'll be able to without having jumping to here conclusions. Use logs, check circumstances, and user reviews to piece together a clear photograph of what’s going on.
Upcoming, sort hypotheses. Question by yourself: What may be triggering this conduct? Have any modifications recently been made to the codebase? Has this issue happened right before less than very similar situation? The purpose is always to narrow down alternatives and establish probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue inside of a controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the reality.
Shell out close awareness to tiny details. Bugs generally hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Lastly, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in complex techniques.
Publish Checks
Writing tests is one of the best solutions to help your debugging skills and All round growth effectiveness. Checks don't just help catch bugs early but additionally serve as a safety net that provides you self confidence when building improvements towards your codebase. A well-tested application is easier to debug because it allows you to pinpoint precisely in which and when a difficulty happens.
Begin with unit tests, which focus on person functions or modules. These small, isolated tests can quickly expose irrespective of whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know wherever to glance, appreciably minimizing time invested debugging. Device checks are Specially valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Following, integrate integration tests and conclusion-to-conclude exams into your workflow. These help make sure several areas of your application do the job collectively easily. They’re significantly handy for catching bugs that take place in complex devices with several components or expert services interacting. If one thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and view your take a look at go when the issue is settled. This tactic makes certain that the identical bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the disheartening guessing game into a structured and predictable approach—encouraging you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous builders report getting the basis of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious perform within the background.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Vitality along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver close to, extend, or do some thing unrelated to code. It may well really feel counterintuitive, Primarily below limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
In short, using breaks is not really a sign of weak point—it’s a sensible strategy. It offers your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It truly is a possibility to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can educate you a thing important in the event you take some time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries when the bug is fixed: What prompted it? Why did it go unnoticed? Could it are actually caught before with better practices like unit tests, code reviews, or logging? The responses often expose blind places in the workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll start to see patterns—recurring issues or popular issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, assisting others stay away from the identical issue boosts staff effectiveness and cultivates a stronger Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce ideal code, but individuals that constantly master from their blunders.
Eventually, Every bug you deal with adds a fresh layer towards your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.